jQuery 연결 방법 1: js파일을 다운로드 서버에 저장하고 연결
보기쉽게 정리되어있는 파일
jquery-3.7.1.min.js(줄바꿈,공백,들여쓰기등을 없앤 압축형태)-난독화 한 것
다운로드 한 뒤에 js폴더에 넣는다.
jQuery 연결 방법 2: CDN(contents Delivery Network)방식: URL를 통해 js 파일 연결
jQuery
What is jQuery? jQuery is a fast, small, and feature-rich JavaScript library. It makes things like HTML document traversal and manipulation, event handling, animation, and Ajax much simpler with an easy-to-use API that works across a multitude of browsers.
jquery.com
다운로드를 들어가서 google CDN을 누른뒤
<script src="https://ajax.googleapis.com/ajax/libs/jquery/3.7.1/jquery.min.js"></script>
를 사용하면 일일히 명시하지 않아도 가능하다.
s01_ready.html
<!DOCTYPE html>
<html>
<head>
<meta charset="UTF-8">
<title>jQuery 연결</title>
<!-- jQuery 연결 방법 1: js파일을 다운로드 서버에 저장하고 연결 -->
<!-- <script type="text/javascript" src="../js/jquery-3.7.1.min.js"></script> -->
<!-- jQuery 연결 방법 2: CDN(contents Delivery Network)방식: URL를 통해 js 파일 연결 -->
<script src="https://ajax.googleapis.com/ajax/libs/jquery/3.7.1/jquery.min.js"></script>
<script type="text/javascript">
//window.onload = function(){};
//문서가 준비 완료되면 매개변수로 전달된 함수를 실행하라는 의미
$(document).ready(function(){
alert('First Ready!!');
});
</script>
</head>
<body>
</body>
</html>
s02_all.html
<!DOCTYPE html>
<html>
<head>
<meta charset="UTF-8">
<title>기본 선택자 - 전체 선택자</title>
<script src="https://ajax.googleapis.com/ajax/libs/jquery/3.7.1/jquery.min.js"></script>
<script type="text/javascript">
//문서가 준비 완료되면 매개변수로 전달된 함수 실행
$(document).ready(function(){
// *: 전체 선택자(문서 객체 선택)
$('*').css('color','red'); //css메서드 : style 처리
});
</script>
</head>
<body>
<h1>장마</h1>
</body>
</html>
s03_tagname.html
<!DOCTYPE html>
<html>
<head>
<meta charset="UTF-8">
<title>기본 선택자 - 태그 선택자, 클래스 선택자, id 선택자</title>
<script src="https://ajax.googleapis.com/ajax/libs/jquery/3.7.1/jquery.min.js"></script>
<script type="text/javascript">
//문서가 준비 완료되면 매개변수로 전달된 함수 실행
$(document).ready(function(){
//태그 선택자
// 속성 속성값
$('span').css('border','3px solid blue');
//클래스 선택자
$('.my').css('border','5px solid red');//my앞에 .을 찍지않으면 태그로 인식한다.
//id 선택자
$('#content').css('background-color','green');
});
</script>
</head>
<body>
<p class="my">jQuery 연습</p>
<div>
<div id = "content">id값이 content인 div tag</div>
</div>
<span>span1</span>
<span class="my">span2</span>
</body>
</html>
p태그와 span태그를 배열로 인식한다.
s04_group.html
<!DOCTYPE html>
<html>
<head>
<meta charset="UTF-8">
<title>그룹 선택자</title>
<script src="https://ajax.googleapis.com/ajax/libs/jquery/3.7.1/jquery.min.js"></script>
<script type="text/javascript">
//문서가 준비 완료되면 매개변수로 전달된 함수를 실행
$(document).ready(function(){
//그룹 선택자
$('h1,p').css('color','orange');
//특정 id 속성을 가지는 태그 선택
$('h3#target').css('color','skyblue');
});
</script>
</head>
<body>
<h1>강물은 흐르고</h1>
<h2>새벽 바람이 차다</h2>
<p>내 눈물도 흐르고</p>
<div>별이 흐른다.</div>
<h3>header-0</h3>
<h3 id="target">header-1</h3>
<h3>header-2</h3>
</body>
</html>
s05_class.html
<!DOCTYPE html>
<html>
<head>
<meta charset="UTF-8">
<title>클래스 선택자 이용</title>
<script src="https://ajax.googleapis.com/ajax/libs/jquery/3.7.1/jquery.min.js"></script>
<script type="text/javascript">
$(document).ready(function(){
$('.item').css('color','orange');
$('h1.item').css('background-color','red');
//두 개의 클래스를 모두 가지는 태그 선택
$('h1.item.select').css('background','blue');//이걸 안쓰면 배경색은 red이다.
});
</script>
</head>
<body>
<h1 class = "item">Header-0</h1>
<h2 class = "item">Header-1</h2>
<h1 class = "item select">Header-2</h1>
<h1 class = "item">Header-3</h1>
</body>
</html>
s06_child.html
<!DOCTYPE html>
<html>
<head>
<meta charset="UTF-8">
<title>자식선택자</title>
<script src="https://ajax.googleapis.com/ajax/libs/jquery/3.7.1/jquery.min.js"></script>
<script type="text/javascript">
$(document).ready(function(){
//자식 선택자
$('body > div').css('border','3px solid red'); //body 밑에있는 div를 선택. 서울은 바로 아래가 아니라 적용이안됨
});
</script>
</head>
<body>
<div>
<ul>
<li>한국</li>
<li>미국</li>
<li>일본</li>
<li>중국</li>
<li>호주</li>
</ul>
<div>서울</div>
</div>
</body>
</html>
s07_descendant.html
<!DOCTYPE html>
<html>
<head>
<meta charset="UTF-8">
<title>후손선택자</title>
<script src="https://ajax.googleapis.com/ajax/libs/jquery/3.7.1/jquery.min.js"></script>
<script type="text/javascript">
$(document).ready(function(){
//후손 선택자
$('body div').css('border','3px solid red'); //body안에 div안에 div도 선택.
});
</script>
</head>
<body>
<div>
<ul>
<li>한국</li>
<li>미국</li>
<li>일본</li>
<li>중국</li>
<li>호주</li>
</ul>
<div>서울</div>
</div>
</body>
</html>
자식 선택자에 '>' 만 제거하고 body와 div 사이 공백만 있으면됨
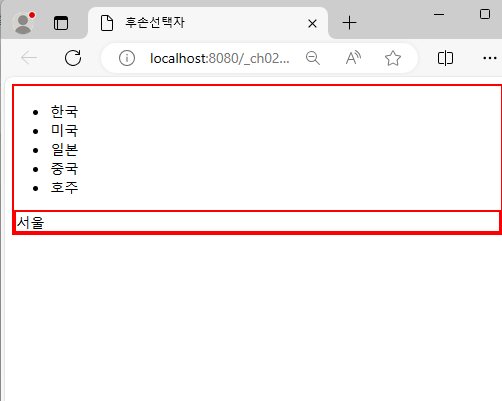
'쌍용교육(JAVA) > jquery' 카테고리의 다른 글
쌍용교육 -jquery수업 42일차 jQuertEvent(2) (0) | 2024.04.17 |
---|---|
쌍용교육 -jquery수업 41일차 jQuertEvent(1) (0) | 2024.04.16 |
쌍용교육 -jquery수업 41일차 jQuerySelector(3) (0) | 2024.04.16 |
쌍용교육 -jquery수업 41일차 jQuerySelector(2) (0) | 2024.04.16 |
쌍용교육 -jquery수업 41일차 jQuerySelector(1) (0) | 2024.04.16 |